Complete "C"
Wednesday, May 13, 2020
C program to make a calculator using the switch statement.
Tuesday, May 12, 2020
check whether a given number is even or odd? If the number is an even number, print it's square.
C program to check whether a given number is even or odd? if the number is even number, print it's square...
#include<stdio.h>
#include<conio.h>
void main()
{
int num, squ;
printf("enter number = ");
scanf("%d",& num);
if(num%2==0)
{
printf("The number is Even");
squ = num*num;
printf("\nsquare of this even number is %d", squ);
}
else
printf("The number is Odd");
getch();
}
Output:
Click Here for, All Important Questions with Answers
Click here for, C Programs
Switch Versus if-else Ladder
Q. What is the difference between the Switch statement and if- else ladder statements?
S.No. |
Switch statement |
If- else-if ladder statements |
|
Instead of using the if-else-if Ladder
control statement, the Switch Statement is available in program C for
Handling multiple choices. In Switch statement three keywords are used
switch, case, and default. we can use integer. constant. but we can not use floating
point and string constants.
|
This
is a type of nesting in which there is an if...else statement in every else
part except the last else part. This type nesting is called the else if
Ladder Statement.
|
|
Syntax: Switch(expression) { case constant 1: statement; break; case constant 2: statement; break; case
constant 3: statement; break; default; statement }
|
Syntax: if(Condition
1)
Statement A; else if(Condition
2)
Statement B; else if(Condition
3)
Statement C;
else if(Condition 4)
Statement C;
else
Statement D;
|
3. |
It’s also called cases control
statement. |
It’s also called loader if
statement.
|
4. |
In switch statements we use
switch keyword. |
Here we use else if keywords.
|
5. |
It is easy to use &
understand. |
It is complex statements.
|
6. |
It can have different cases for
different conditions. |
But there is no different cases
for different conditions. |
7. |
In this statement, we can not use
relational & logical operators. |
In this we can use relational
& logical statements.
|
8. |
#include<stdio.h> #include<stdio.h> void main() { int ch; printf("\n1.
press ch=1 for monday. \n2. press ch=2 for tuesday.\n3. press ch=3 for
wednesday. \n4.press ch=4 for thursday. \n5.press ch=5 for friday.\n6.press
ch=6 for saturday. \n7. press ch=7 for sunday.");
printf("\n
enetr your choice =");
scanf("%d", &ch); switch(ch) {
case 1:
printf("today is monday");
break;
case 2:
printf("today is tuesday");
break;
case 3:
printf("today is wednesday");
break;
case 4:
printf("today is thursday");
break;
case 5:
printf("today is friday");
break;
case 6:
printf("today is saturday");
break;
case 7:
printf("today is sunday");
break;
default:
printf("wrong choice Try Again!!!"); }
getch();
}
Output: |
#include<stdio.h> #include<stdio.h> void main() { int
ch;
printf("\n1. press ch=1 for monday. \n2. press ch=2 for tuesday.\n3.
press ch=3 for wednesday. \n4.press ch=4 for thursday. \n5.press ch=5 for
friday.\n6.press ch=6 for saturday. \n7. press ch=7 for sunday.");
printf("\n enetr your choice =");
scanf("%d", &ch);
if(ch==1) {
printf("today is monday"); } else
if(ch==2) {
printf("today is tuesday"); } else
if(ch==3) {
printf("today is wednesday"); } else
if(ch==4) {
printf("today is thursday"); } else
if(ch==5) {
printf("today is friday"); } else
if(ch==6) {
printf("today is saturday"); } else
if(ch==7) {
printf("today is sunday"); }
getch(); } Output:
|
Monday, May 11, 2020
Check whether the Alphabet is a Vowel or Consonant
Sunday, May 10, 2020
C Program to Print Day Name of Week using Switch Statements
Saturday, May 9, 2020
The Switch Statement
Friday, May 8, 2020
What is Scope of Global and Local Variable in C ?
Syntax:
function(a, b)
int a, b;
{
int x, y; /*Local Variable*/
/*body of the function*/
}
Example:
#include<stdio.h>
#include<conio.h>
void main()
{
int a, b, sum; /*Local Variable*/
/* Printf() outputs the values to the screen whereas scanf() receive them from the keyboard. and & is a address used in scanf(). */
printf("enter first no=");
scanf("%d", & a);
printf("enter second no=");
scanf("%d", & b);
sum= a+b;
printf("Sum of two number is =%d",sum);
getch();
}
Output:
Global Variable:- Global Variable are defined outside the main() function block. All functions in the program can access and modify global variables. Its's very useful if it is to be used by many functions in the program. this variables are automatically initialized by 0 at the time of declaration.
Syntax:
int x, y; /*Global Variable*/
main()
{
x=5;
function1();
}
function1();
{
int sum;
sum=x+y;
}
Example:
#include<stdio.h>
#include<conio.h>
int sum; void main()
{
int a, b; /*Local Variable*/
/* Printf() outputs the values to the screen whereas scanf() receive them from the keyboard. and & is a address used in scanf(). */
printf("enter first no=");
scanf("%d", & a);
printf("enter second no=");
scanf("%d", & b);
sum= a+b;
printf("Sum of two number is =%d",sum);
getch();
}
Output:
Click here, for All Important Questions
-
#include<stdio.h> #include<stdio.h> void main() { int ch; printf("\n1. press ch=1 for monday. \n2. press ch=2 ...
-
* The if-else statement Q. Request the user to inputs an integer and, if the number is divisible by two, divides it by two, otherwi...
-
Instead of using the if-else-if Ladder control statement, the Switch Statement is available in program C for Handling multiple choices. In ...
C program to make a calculator using the switch statement.
/*perform arithmetic calculation on integers in C language*/ #include<stdio.h> #include<conio.h> void main() { char op; ...
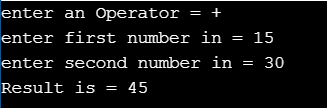